Day 11 #100DaysOfCode
Hôm này mình tìm hiểu tiếp về Migrations trong Laravel 5.8 ở loạt bài viết trước mình đã tìm hiểu sơ qua về Laravel 5.8 rồi, chắc cũng hiểu sơ sơ rồi
Trong Laravel theo mình thì mình thích thằng Migrations này vì nó cho phép ta thiếp lập cấu hình Table tuy ý, liên kết table(relationship). Nếu các bạn có xem qua ASP.NET MVC 5, ASP.NET CORE , thì ASP.NET cũng cho phép người dùng tạo migrations để xây dụng cấu trúc database
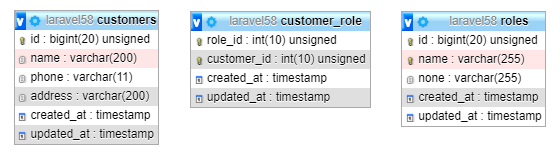
php artisan make:migration create_customers_table //or php artisan make:migration create_customers_table --table=customers //or php artisan make:migration create_customers_table --create=customers
--create=customers : khi gắn thêm vào câu lệnh , ta sẽ được cấu trúc điền sẵn để tạo table
--table=customers : nếu bạn dùng tính năng này, bạn sẽ nhìn thấy code, cấu trúc sửa đổi của table, đối với table hiện có trong database
Sao khi bạn chạy thành công bạn sẽ được file migration trong đường dẫn thư mục này App\database\migrations\ sẽ có cấu trúc giống như thế này!
<?php use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreateCustomersTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('customers', function (Blueprint $table) { $table->bigIncrements('id'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('customers'); } }
+ Tạo table mới từ trong migration, ta dùng phương thức create(), đầu tiền là tham số tên của table "name", thứ hai là ta cần xác định cột chứa dữ liệu
Schema::create('customers', function (Blueprint $table) { });
+ Tạo cột mới vào table hiện có , ta phải sử dụng Blueprint
Schema::create('customers', function (Blueprint $table) { $table->bigIncrements('id'); $table->timestamps(); });
+ Nếu bạn chỉnh sửa table, cần thêm một cột vào sau một cột khác bạn có thể dùng cách sau
Schema::table('customers', function (Blueprint $table) { $table->string('email')->nullable()->after('name'); });
+ Xóa table ta sử dụng hàm dropIfExists()
Schema::dropIfExists('customers');
+ Chỉnh sửa cột , ví dụ cột name có chiều dài 255, nhưng giờ mình đổi lại 100, ta thực hiện thao tác sau
Schema::table('users', function (Blueprint $table) { $table->string('name', 100)->change(); });
+ Chỉnh sửa tên cột, ví dụ: "des" ->"description"
Schema::table('contacts', function (Blueprint $table) { $table->renameColumn('des', 'description'); });
+ Để drop một cột, ta dùng cách sau
Schema::table('contacts', function (Blueprint $table) { $table->dropColumn('votes'); });
+ Thiếp lặp khóa chính primary
$table->primary('primary_id');
Nếu bạn đang dùng phương thức increments() or bigIncrements() thì bạn khỏi quan tâm tới primary(), vì trong increments() or bigIncrements() nó sẽ tạo khóa auto cho mình
Schema::create('customers', function (Blueprint $table) { $table->bigIncrements('id'); $table->timestamps(); });
+ Xóa khóa primary
$table->dropPrimary('primary_id');
+ Lặp chỉ mục indexes : dùng để tránh trùng lặp dữ liệu, ở đây mình muốn email được lặp chỉ mục
$table->unique('email');
+ xóa chỉ mục indexes:
$table->dropUnique('email'); // or $table->dropIndex(['email', 'amount']);
+ Thêm khóa ngoại
$table->foreign('user_id')->references('id')->on('users'); //or $table->foreign('role_id')->references('id')->on('roles')->onDelete('cascade');
+ Xóa khóa ngoại
$table->dropForeign(['role_id']);
Ta có thể xem các phương thức trong migration mà ta có thể tạo cột cho dữ liệu table https://laravel.com/docs/5.8/migrations
Okay, giờ cần thiếp lập file migration chúng ta vừa tạo, để chạy lệnh migration tạo table
+ 2021_01_09_014041_create_customers_table.php trong thư mục App\database\Migrations mình cần chỉnh lại function up() như sau:
Schema::create('customers', function (Blueprint $table) { $table->bigIncrements('id'); $table->string('name',200); $table->string('phone',11); $table->string('address',200); $table->timestamps(); });
+ 2021_01_09_013922_create_roles_table.php
Schema::create('roles', function (Blueprint $table) { $table->bigIncrements('id'); $table->string('name')->unique(); $table->string('none'); $table->timestamps(); });
+ 2021_01_09_015308_create_customer_role_table.php : liên kế relationship giữa table "customers","roles"
Schema::create('customer_role', function (Blueprint $table) { $table->integer('role_id')->unsigned(); $table->integer('customer_id')->unsigned(); $table->primary(['customer_id','role_id']); $table->timestamps(); });
Code trên mình liên kết Many-to-Many giữa 2 table "customers","roles"
+ Khách hàng(customers) sẽ có nhiều quyền(rolers)
+ Một quyền(roles) sẽ có nhiều khách hàng(customers)
Okay giờ mình chạy lệnh thực thi migration như sau
php artisan migrate
Nếu không có lỗi gì bạn sẽ được hình như dưới đây
Trong migration có các lệnh dưới đây, bạn có thể tìm hiểu thêm
- migrate:install
- migrate:reset
- migrate:refresh
- migrate:fresh
- migrate:rollback
- migrate:status
Okay vậy xong ngày 11 #100DaysOfCode